

CODE
/*
Standalone Sketch to use with a Arduino UNO and a
Sharp Optical Dust Sensor GP2Y1010AU0F
Blog: http://arduinodev.woofex.net/2012/12/01/standalone-sharp-dust-sensor/
Code: https://github.com/Trefex/arduino-airquality/
For Pin connections, please check the Blog or the github project page
*/
#include <SPI.h>
#include <SD.h>
//const int chipSelect = 4;
int measurePin = 5;
int ledPower = 8;
int ledlow1 = 5;
int ledmed1 = 3;
int ledhigh2 = 7;
int ledlow2 = 4;
int ledmed2 =2;
int ledhigh1 = 6;
int samplingTime = 280;
int deltaTime = 40;
int sleepTime = 9680;
float voMeasured=0.0;
float calcVoltage=0.0;
float dustDensity=0.0;
void setup() {
Serial.begin(9600);
pinMode(ledlow1, OUTPUT);
pinMode(ledmed1, OUTPUT);
pinMode(ledhigh1, OUTPUT);
pinMode(ledlow2, OUTPUT);
pinMode(ledmed2, OUTPUT);
pinMode(ledhigh2, OUTPUT);
pinMode(ledPower, OUTPUT);
delay(3000);
}
void loop() {
digitalWrite(ledlow1,LOW);
digitalWrite(ledlow2,LOW);
digitalWrite(ledmed1,LOW);
digitalWrite(ledmed2,LOW);
digitalWrite(ledhigh1,LOW);
digitalWrite(ledhigh2,LOW);
digitalWrite(ledPower, LOW); // power on the LED
delayMicroseconds(samplingTime);
voMeasured = analogRead(measurePin); // read the dust value
delayMicroseconds(deltaTime);
digitalWrite(ledPower, HIGH); // turn the LED off
delayMicroseconds(sleepTime);
calcVoltage = voMeasured * (5.0 / 1024);
dustDensity = (0.17 * calcVoltage - 0.1) * 1000;
if (dustDensity<0) {
dustDensity = 0;
}
if (dustDensity>0){
digitalWrite(ledlow1,HIGH);
}
if (dustDensity>40){
digitalWrite(ledlow2,HIGH);
}
if (dustDensity>60){
digitalWrite(ledmed1,HIGH);
}
if (dustDensity>100){
digitalWrite(ledmed2,HIGH);
}
if (dustDensity>200){
digitalWrite(ledhigh1,HIGH);
}
if (dustDensity>300){
digitalWrite(ledhigh2,HIGH);
}
Serial.print("Raw: ");
Serial.print(voMeasured);
Serial.print("; - Voltage: ");
Serial.print(calcVoltage);
Serial.print("; - Dust Density [ug/m3]: ");
Serial.print(dustDensity);
Serial.println(";");
delay(1000);
}
Sharp's GP2Y1010AU0F is an optical air quality sensor, or may also known as optical dust sensor, is designed to sense dust particles. An infrared emitting diode and a phototransistor are diagonally arranged into this device, to allow it to detect the reflected light of dust in air. It is especially effective in detecting very fine particles like cigarette smoke, and is commonly used in air purifier systems.
Wood dust in the workshop is one of the major hazards that needs addressing. A link below is to a site that details the different woods and their respective hazards although in general terms harder (and more interesting woods) tend to be the most dangerous however all wood dust is to be avoided where possible.
This page shows how a fairly simple but very accurate dust meter can be made using an Arduino and an easily available Sharp dust sensor.
The graph above to the right taken from the specifications of the Sharp sensor shows a substantial linear section that makes a simple equation possible (found from the Instructables website) to convert the output voltage to the estimated dust density.
I did think of a numeric output but for ease decided to use a series of leds to display the increasing dusty and dangerous density. When the red leds begin to be lit then that is the time to exit the workshop leaving doors and windows open!!
I used an old computer fan to draw air in and through the detector.
In use perhaps it is perhaps of less value than I imagined as the dust varies a lot around the workshop and so the position of the box is important. Ideally I guess I ought to be suspending it around my neck but that does not seem practical. What was useful however was to make dust on the lathe and hold the device close by to get a sense of when the level of dust hit the maximum safe limit (this was when the first of the red leds lit)
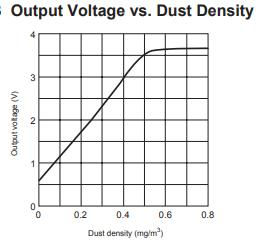
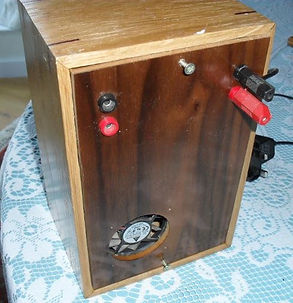

CODE 2
/*
Standalone Sketch to use with a Arduino UNO and a
Sharp Optical Dust Sensor GP2Y1010AU0F
Blog: http://arduinodev.woofex.net/2012/12/01/standalone-sharp-dust-sensor/
Code: https://github.com/Trefex/arduino-airquality/
For Pin connections, please check the Blog or the github project page
*/
#include <SPI.h>
#include <SD.h>
//const int chipSelect = 4;
int measurePin = 5;
int ledPower = 8;
int ledlow1 = 5;
int ledmed1 = 3;
int ledhigh2 = 7;
int ledlow2 = 4;
int ledmed2 =2;
int ledhigh1 = 6;
int samplingTime = 280;
int deltaTime = 40;
int sleepTime = 9680;
float voMeasured=0.0;
float calcVoltage=0.0;
float dustDensity=0.0;
void setup() {
Serial.begin(9600);
pinMode(ledlow1, OUTPUT);
pinMode(ledmed1, OUTPUT);
pinMode(ledhigh1, OUTPUT);
pinMode(ledlow2, OUTPUT);
pinMode(ledmed2, OUTPUT);
pinMode(ledhigh2, OUTPUT);
pinMode(ledPower, OUTPUT);
delay(3000);
}
void loop() {
digitalWrite(ledlow1,LOW);
digitalWrite(ledlow2,LOW);
digitalWrite(ledmed1,LOW);
digitalWrite(ledmed2,LOW);
digitalWrite(ledhigh1,LOW);
digitalWrite(ledhigh2,LOW);
digitalWrite(ledPower, LOW); // power on the LED
delayMicroseconds(samplingTime);
voMeasured = analogRead(measurePin); // read the dust value
delayMicroseconds(deltaTime);
digitalWrite(ledPower, HIGH); // turn the LED off
delayMicroseconds(sleepTime);
calcVoltage = voMeasured * (5.0 / 1024);
dustDensity = (0.17 * calcVoltage - 0.1) * 1000;
if (dustDensity<0) {
dustDensity = 0;
}
if (dustDensity>0){
digitalWrite(ledlow1,HIGH);
}
if (dustDensity>40){
digitalWrite(ledlow2,HIGH);
}
if (dustDensity>60){
digitalWrite(ledmed1,HIGH);
}
if (dustDensity>100){
digitalWrite(ledmed2,HIGH);
}
if (dustDensity>200){
digitalWrite(ledhigh1,HIGH);
}
if (dustDensity>300){
digitalWrite(ledhigh2,HIGH);
}
Serial.print("Raw: ");
Serial.print(voMeasured);
Serial.print("; - Voltage: ");
Serial.print(calcVoltage);
Serial.print("; - Dust Density [ug/m3]: ");
Serial.print(dustDensity);
Serial.println(";");
delay(1000);
}